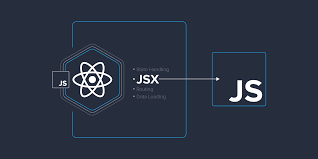
React uses JSX (JavaScript XML) to create UI elements efficiently. JSX provides a syntax similar to HTML, making it easy to structure and render components. In this blog, we will explore the following topics:
- Rendering JSX Elements
- Nesting Elements in JSX
- Using Fragments (
<>...</>
)
Rendering JSX Elements
JSX allows us to write elements inside JavaScript code and render them efficiently within the DOM using React. Each JSX element is compiled into React.createElement()
calls under the hood.
Example:
import React from 'react'; import ReactDOM from 'react-dom/client'; const element = <h1>Hello, World!</h1>; const root = ReactDOM.createRoot(document.getElementById('root')); root.render(element);
Explanation:
- The JSX element
<h1>Hello, World!</h1>
is assigned to theelement
variable. ReactDOM.createRoot(document.getElementById('root'))
creates a root reference..render(element)
attaches the JSX element to the DOM.
Nesting Elements in JSX
JSX allows nesting elements, enabling the creation of complex UIs with hierarchical structures.
Example:
const nestedElements = ( <div> <h1>Welcome to React</h1> <p>This is a paragraph inside a div.</p> <ul> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul> </div> ); root.render(nestedElements);
Explanation:
- The
div
acts as a wrapper. - It contains an
h1
, ap
, and aul
withli
elements. - This structure is directly passed to
.render()
.
Using Fragments (<>...</>
)
JSX requires a single parent element. When multiple elements need to be returned without introducing extra markup, React Fragments are used.
Example Without Fragment:
const invalidJSX = ( <h1>Hello</h1> <p>This will cause an error</p> );
The above code will result in an error because JSX must return a single element.
Using React Fragments:
const validJSX = ( <> <h1>Hello</h1> <p>This will work correctly</p> </> ); root.render(validJSX);
Explanation:
<>...</>
acts as an invisible wrapper.- It prevents unnecessary
div
nesting, improving performance and maintaining a clean DOM structure.
Conclusion
JSX simplifies UI development in React by allowing us to write HTML-like structures inside JavaScript. We explored:
- Rendering JSX elements using
ReactDOM.createRoot().render()
. - Nesting elements to create structured UIs.
- Using fragments (
<>...</>
) to avoid unnecessary wrapper elements.
Mastering JSX is the first step toward building dynamic and scalable React applications!
Leave a Comment